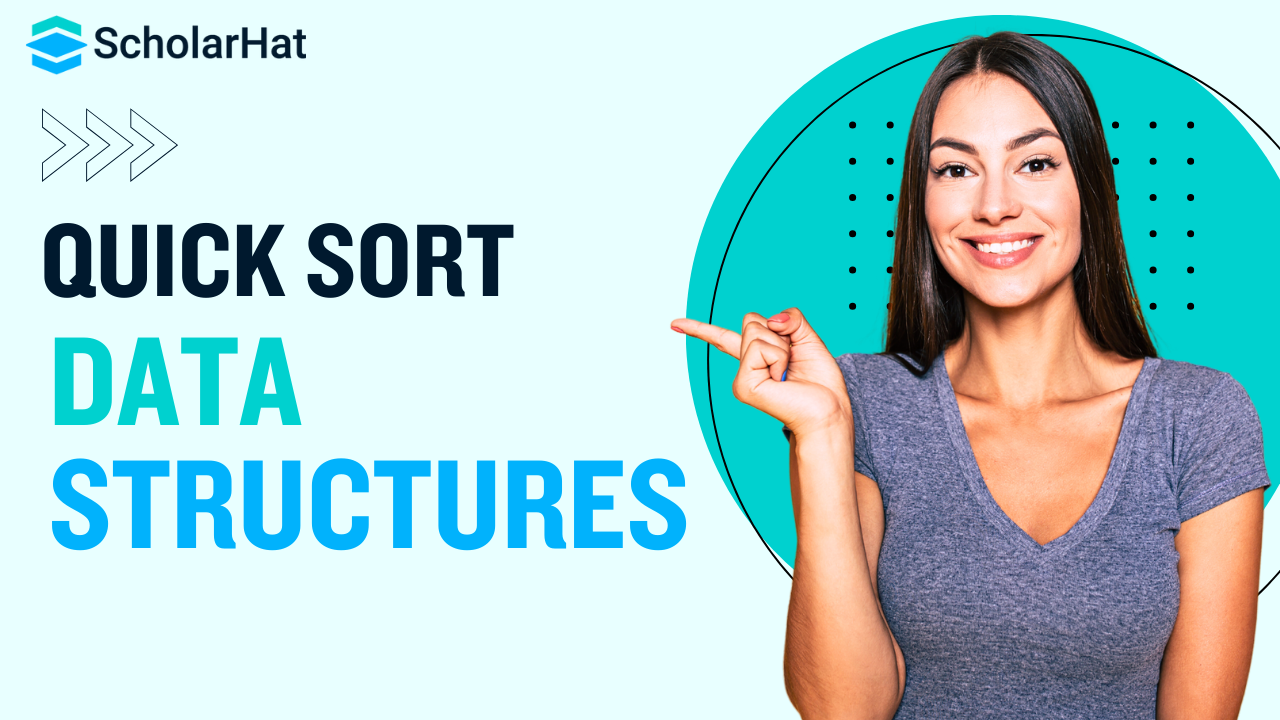
Quick Sort in Data Structure: A Swift and Efficient Sorting Algorithm
Introduction
In the realm of data structures and algorithms, quick sort stands out as a versatile and efficient sorting algorithm. This article aims to provide a comprehensive guide to quick sort, exploring its features, benefits, and how it can revolutionize the way we sort data.
Understanding Quick Sort
Quick sort in Data Structure is a comparison-based sorting algorithm that follows the divide-and-conquer approach. It works by selecting a pivot element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted using the same process until the entire array is sorted.
Advantages of Using Quick Sort
-
Swift Sorting: Quick sort is known for its speed and efficiency. It has an average-case time complexity of O(n log n), making it one of the fastest sorting algorithms available.
-
In-Place Sorting: Quick sort performs sorting in-place, meaning it doesn’t require additional memory to store temporary arrays. This makes it memory-efficient and ideal for scenarios with limited memory resources.
-
Adaptability: Quick sort adapts well to different data distributions. It performs exceptionally well on average and random data sets, making it suitable for a wide range of applications.
Getting Started with Quick Sort
To implement quick sort in Data Structure we need to understand the basic steps involved:
Step 1: Selecting a Pivot
The pivot element is crucial in quick sort as it determines the partitioning of the array. It can be chosen in various ways, such as selecting the first element, the last element, or a random element from the array.
Step 2: Partitioning the Array
Once the pivot is selected, we partition the array into two sub-arrays, one containing elements smaller than the pivot and the other containing elements larger than the pivot. This is achieved by comparing each element with the pivot and swapping them accordingly.
Step 3: Recursive Sorting
After partitioning, we recursively apply the same process to the sub-arrays until the entire array is sorted. This is done by selecting a new pivot for each sub-array and repeating the partitioning and sorting steps.
Advanced Techniques in Quick Sort
Quick sort in Data Structure offers various advanced techniques to enhance its performance and efficiency:
Randomized Quick Sort
Randomized quick sort improves the algorithm’s performance by randomly selecting the pivot element. This helps avoid worst-case scenarios where the array is already sorted or nearly sorted, which can lead to poor partitioning and inefficient sorting.
Three-Way Partitioning
In some cases, quick sort can encounter performance issues when dealing with arrays that contain many duplicate elements. Three-way partitioning addresses this by partitioning the array into three sub-arrays: one with elements less than the pivot, one with elements equal to the pivot, and one with elements greater than the pivot.
Hybrid Sorting
Hybrid sorting techniques combine quick sort in Data Structure with other sorting algorithms to further optimize performance. For example, quick sort can be used for partitioning, and then insertion sort can be applied to the smaller sub-arrays, taking advantage of insertion sort’s efficiency for small-sized arrays.
Conclusion
Quick sort is a powerful and efficient sorting algorithm that allows swift sorting of data. With its divide-and-conquer approach and ability to adapt to various data distributions, quick sort proves to be a reliable choice for sorting tasks. By understanding the concepts and techniques discussed in this article, developers can harness the power of quick sort and simplify their sorting processes. Whether it’s sorting large datasets, implementing search algorithms, or organizing data structures, quick sort provides a swift and efficient solution.