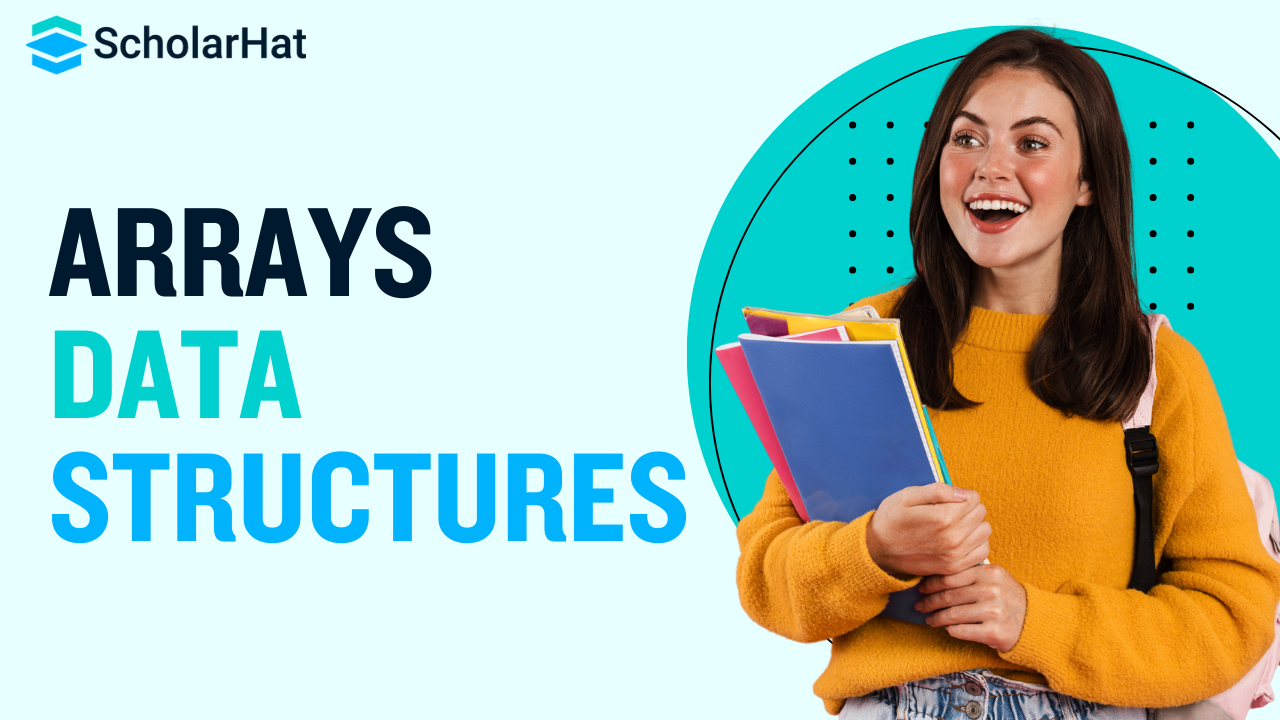
Array Data Structure: Exploring the Building Blocks of Efficient Data Storage
Introduction
In the vast world of data structures, the array data structure stands as one of the fundamental and versatile tools for efficient data storage. This article aims to provide a comprehensive guide to the array data structure, exploring its features, benefits, and how it can revolutionize the way we handle and manipulate data.
Understanding the Array Data Structure
An array is a fixed-size collection of elements of the same data type, stored in contiguous memory locations. It provides a convenient way to store and access data, as each element can be accessed directly through its index. Arrays can be of various dimensions, ranging from one-dimensional arrays (vectors) to multi-dimensional arrays (matrices).
Advantages of Using Arrays
-
Efficient Access: Arrays offer constant time complexity for accessing elements, as they can be accessed directly through their index. This allows for efficient retrieval and manipulation of data.
-
Memory Efficiency: Arrays in Data Structure provide efficient memory usage, as they allocate contiguous memory locations. This makes arrays ideal for scenarios where memory resources are limited.
-
Versatility: Arrays can store elements of any data type, allowing for the storage of integers, floating-point numbers, characters, or even objects. This versatility makes arrays suitable for a wide range of applications.
Getting Started with Arrays
To start using arrays, we need to understand the basic operations involved:
Step 1: Declaring an Array
To declare an array, we specify its data type and size. For example, to declare an array of integers with a size of 10, we can write: int myArray[10];.
Step 2: Initializing an Array
Arrays can be initialized either during declaration or later in the code. During initialization, we assign values to each element of the array. For example, int myArray[5] = {1, 2, 3, 4, 5};.
Step 3: Accessing Array Elements
Array elements can be accessed by their index. The index starts from 0 for the first element and increments by 1 for each subsequent element. For example, int element = myArray[2]; will assign the value of the third element to the variable element.
Advanced Operations on Arrays
Arrays offer a range of advanced operations to enhance their functionality:
Searching
-
Linear Search: Linear search is a simple and straightforward method for finding an element in an array. It involves traversing the array element by element until the desired element is found or the end of the array is reached.
-
Binary Search: Binary search is a more efficient search algorithm for sorted arrays. It follows a divide-and-conquer approach, repeatedly dividing the array in half until the desired element is found or the search range is reduced to zero.
Sorting
-
Selection Sort: Selection sort is a simple sorting algorithm that repeatedly selects the minimum element from the unsorted part of the array and swaps it with the element at the beginning of the sorted portion.
-
Insertion Sort: Insertion sort builds the final sorted array one element at a time by inserting each element into its correct position within the already sorted portion of the array.
Dynamic Arrays
Dynamic arrays, also known as resizable arrays or vectors, allow for the dynamic allocation and resizing of arrays. They provide flexibility in terms of size, allowing arrays to grow or shrink based on the requirements of the program.
Conclusion
The array data structure serves as a fundamental building block for efficient data storage and manipulation. With its efficient access, memory efficiency, and versatility, arrays prove to be invaluable in various programming scenarios. By understanding the concepts and techniques discussed in this article, developers can harness the power of arrays and optimize their data handling processes. Whether it’s storing and accessing large datasets, implementing search algorithms, or organizing data structures, arrays provide a solid foundation for efficient and effective programming.